npm i chaperone
import { Chaperone, ChaperoneStep, Trigger } from "chaperone";
it is the basic usage of chaperone.
Don't forget to assign isDone at the last step or else it will throw exception.
it is use to make multiple and different id steps in single ChaperoneStep.
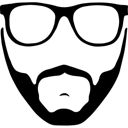
you can create multiple guide in single Chaperone and you can chain another ID after done or skip.
you must be extra careful when using onDone and onSkip in Chaperone component. especially if the component is having some sort of state. or else it will be called infinitely and will cause error in your app. as much as posible use hook useCallback without array dependency to prevent some sort of error any further.
you can create and append gestures.
API
It is the chaperone.
Property Property | Type Type | Default Default | Description Description |
---|---|---|---|
autoTrigger | boolean | false | When true, it will be triggered immidiately without clicking the trigger |
id | string | - | It is the ID of the autoTrigger |
onSkip | function | - | It will be executed if the children ChaperoneStep is skipped. you must be extra careful when using onSkip. especially if the component is having some sort of state. or else it will be called infinitely and will cause error in your app. as much as posible use hook useCallback without array dependency to prevent some sort of error any further. |
onDone | function | - | It will be executed when the children ChaperoneStep is done. you must be extra careful when using onDone. especially if the component is having some sort of state. or else it will be called infinitely and will cause error in your app. as much as posible use hook useCallback without array dependency to prevent some sort of error any further. |
waitSearchIndex | number | 50 | It is the waiting time when searching indexes |
children | element | - | A children element to render. |
It is the chaperone step.
Property Property | Type Type | Default Default | Description Description |
---|---|---|---|
id | string | - | It is use to identify which set of step will be trigger since you can use multiple set of steps to trigger inside Chaperone. |
index | number | - | It is use to determine on what index should this component will be pop. |
message | string | element | - | It is the message of the popover. |
placement | 'top' | 'bottom' | 'left' | 'right' | 'top-left' | 'top-right' | 'bottom-left' | 'bottom-right' | 'left-top' | 'left-bottom' | 'right-top' | 'right-bottom' | bottom-right | It is the placement of the popover. |
duration | miliseconds|number | 200 | It the duration of the transitions. |
navigation | number | 0 | It is use to show the navigation and how many bullets will be render. most commonly the number of your set steps. when 0, it will be hidden |
children | element | - | It will be highligthened target of the step. |
autoScrollDelay | miliseconds | number | 150 | It is the delay when chasing the next or previous step viewport after triggered. |
find | string | function | - | It use to select element within or outside the children. |
allowSkip | boolean | true | When false, the skip button will be hidden. |
allowBack | boolean | true | When false, the back step button will be hidden. |
allowNext | boolean | true | When false, the next step button will be hidden. |
allowNextOnClick | boolean | false | When true, the next step is allowed to be triggered by hitting the highlightened element. |
allowNextOnType | boolean | false | When true, the next step is allowed to be triggered by typing the highlightened form input element. |
isDone | boolean | false | When true, the done button will be shown, and it is use to tell chaperone that it is the last step. it is required to put it one your last steps on every set or it will bring some error. |
isDoneOnClick | boolean | false | When true, done is allowed to be triggered by clicking the highlightened element. |
backToIndex | number | - | It is use to jump back a certain index when clicking the back button. |
nextToIndex | number | - | It is use to jump on a certain index when triggering all the next event. |
onNext | function | - | It will be executed when you triggered all the next events. when you return false it will be prevented to go next. |
onBack | function | - | It will be executed when you clicking the back button. when you return false it will prevented you to go back. |
onSkip | function | - | It will be executed when you clicking the skip button. when you return false it will prevented you to skip. |
onDone | function | - | It will be executed when you triggered all the done events. when you return false it will prevented you to done. |
onLoad | function | - | It will be executed when the entering step. |
onUnLoad | function | - | It will be executed when leaving step. it is use to cleanup your onLoad messes. |
gesture | element | function | - | It will allow you to render a gestures. |
background | object | {} | It allows you to customize the styles of the backdrop, highlight, and gesture. |
propertyMutation | function | - | It allows you to make a multiple steps by providing you the currentId and index, and using the defaultProps to get the pre-assigned props. |
neumorphism | boolean | false | When true, it change the style of the popover. |
hideEvidence | boolean | true | When false, it will not re-render when find matches on every events and will not hide the wrapper <div/> to the children. |
It is the trigger to the chaperone.
Property Property | Type Type | Default Default | Description Description |
---|---|---|---|
as | elementType | <a> | You can use a custom element type for this component. |
label | string|element | - | It is the label of the trigger. |
id | string | - | It is use to determine which set will be open. |
index | number | 0 | It is use to determine which index of the set will be open. |
allowTransition | boolean | false | When true, it allows you to transition effect the highlight. |